
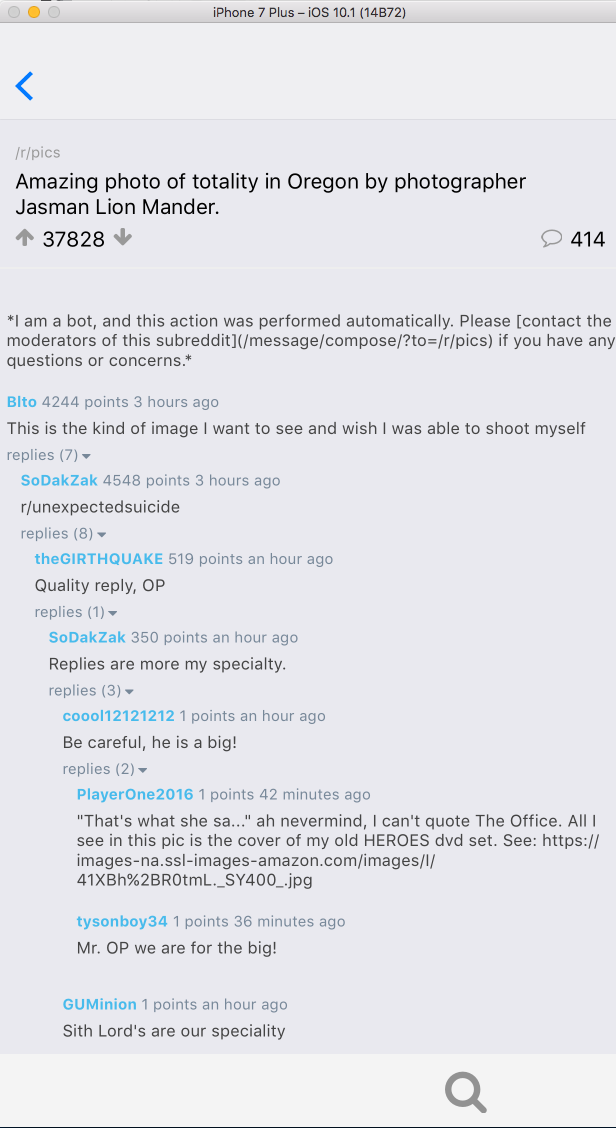

Introduction
This won’t be a full on tutorial, but rather a walk through on how I created a reddit reader using expo, styled-components, and redux saga. It will go over my file structure, navigation, state configuration, and using styled-components to create a more readable component structure.
Repo is here: https://github.com/jm90m/expo-reddit-reader, to run the project simply clone the project and open with Expo. (Get expo here: http://expo.io/)
Features included:
- View frontpage posts
- Infinite scroll on frontpage posts
- View lists of subreddits
- View subreddits posts
- View individual post with comments
Note: Right now clicking on the post image preview, goes to the comments page rather than the actual source of the post. You can easily change it to go to the post source, but I just did it this way because I was too lazy to click on the little comments icon to go to the comments page.
File Structure
I tend to keep all my files in the /src
directory and I use a babel plugin for aliases (npm install — save-dev babel-plugin-module-alias
) to set the project root to the /src
folder, that way imports look cleaner. (Check my .babelrc
for configuration)
Here are the following top-level folders in /src/:
api
— holds file that makes the requests to api.components
— common components that will be used throughout this app.screens
— different screens that the user will navigate to.state
— holds the store configuration as well as all actions/reducers/sagas.constants
— keep reusable style constants such as colors in here, where it is organized by color hue then color name.util
— where I store all helper files, in this case the reddit-data-parser that is used to parsed comments & grab the preview image.
Navigation
The navigation configuration is all held in App.js
. The main navigation is a TabNavigator
and it holds 2 children which are both StackNavigators
. We first have the Feed StackNavigator
, and in it includes the FeedScreen
and CurrentPostScreen
. The FeedScreen
just displays the list of posts while the CurrentPostScreen
is used to display the comments of that particular post. Rather than creating a custom navigator, I simply just used a StackNavigator as it is fairly straightforward to use and it provides several options that you can utilize without any further customization such as the back button or setting the header title. In the FindSubredditScreen
StackNavigator, I have the FindSubredditScreen
, which displays a list of subreddits that the user can view. The SubredditFeedScreen
just displays posts from the clicked subreddit from the FindSubredditScreen
and the CurrentPostScreen displays the list of comments from the post selected from the SubredditFeedScreen
. Rather than creating a custom navigator, I simply just used a StackNavigator as it is fairly straightforward to use and it provides several options that you can utilize without any further customization such as the back button or setting the header title.
State Configuration
Actions
In my action-types file, I like to create a helper function called createAsyncActionType, which just returns an object that has 3 parameters REQUESTED, SUCCESS, ERROR. I use this helper for all my async actions, rather than having separate action types such as FETCH_FEED_SUCCESS, FETCH_PEED_REQUESTED, FETCH_FEED_ERROR
, I simple just have FETCH_FEED = createAsyncActionType(‘fetch_feed’)
and I can use this in my action creators and reducers calling FETCH_ FEED.REQUESTED, FETCH_FEED.SUCCESS, FETCH_FEED.ERROR
Using Redux Saga
I have 3 different sagas, the current-post-saga, feed-saga, and subreddit-saga
, where all the watchers from these 3 sagas get added to the root saga. I like to organize each saga as having a worker sagas and a watcher sagas. The worker sagas, does the actual calling of the API and dispatching the appropriate actions. While the watcher sagas get exported to the root saga, where they listen to dispatched actions.
Styled-Components
With styled-components I was able to create a more readable component structure by providing more context to the actual components. For example in components/comments/comment.js,
I have several child components:

Each child component has a name to it that helps provide more context to what each component is actually used for. In the render method, I combine all child components together:

Conclusion
Repo is here: https://github.com/jm90m/expo-reddit-reader
This was just a simple reddit reader, that I was quickly able to hack together on my spare time. I would like to add more features / cleanup existing features, but time is an issue. For those learning React Native, feel free to fork the project and try adding some features, could help with the process of learning React Native.
I can’t thank the team at Expo enough, there tool makes creating React Native applications more smoother, and way better than using vanilla React Native. They’ve done an amazing job at including all the frameworks needed to quickly create an app in React Native. Also I would like to thank the team at Styled-Components and Redux-Saga, both frameworks are well made and I haven’t found any issues with them. I use styled-components at work in production but for the web. Hoping to use styled-components in my React Native production apps in the future though. Redux-Saga may seem daunting to learn at first, since there are so many concepts to learn, but it is worth the extra time and effort to learn as it provides a more cleaner structure than Redux-Thunk for your async calls, especially when it comes to more complicated async calls. (We recently had to switch from Redux-Thunk to Redux-Saga at my work, since all our async calls were getting too complicated and unreadable). Also I would like to thank this github project (https://github.com/akveo/react-native-reddit-reader), for the help with learning how to use the reddit api, parsing out comments/images, structuring and styling components especially the comments section, and many more.