CSS Tips and Tricks Using Pseudo-Class
We know that CSS Pseudo-Class is very helpful in styling a special state of the selected element(s). Like changing or adding a style when the button is clicked or hovered. Cool Right? But that’s not it, we can do a lot of cool things using CSS Pseudo-Class. In this article, I will cover some of Pseudo-Class and share some tips and tricks that might be helpful and interesting.
:Hover

.hover-target:hover + .hover-element{
opacity: 1;
transform: translateX(0);
}.hover-element{
border-radius: 5px;
background: #c0392b;
margin: 0px 10px;
text-align: center;
padding: 5px 10px;
opacity: 0;
transform: translateX(40px);
transition: all 1.5s ease;
}<div class="content">
<div class="hover-target">HOVER ME</div>
<div class="hover-element">I am hovering</div>
</div>
When we hover on .hover-target
, it will target its Adjacent sibling +
which is .hover-element
and it will trigger the style on .hover-target:hover + .hover-element
that will change its opacity
and transform
of the .hover-element
while the mouse pointer is hovering on .hover-target
.
You can also use general sibling ~
to target multiple sibling elements.
Note: We are using CSS Transitions to create a smooth animation when changing the CSS properties.
Rather that creating an extra html element and targeting its adjacent sibling, when can use ::after Pseudo-elements for better SEO performance and accessibility. ( thanks Daniel Mittleman for pointing this out. ) This way, we are using only one html element and adding the additional content using :after
.
Note: that you need to specify the
content
property or make it emptycontent:''
, so that it will be visible on the page.
.hoverPE-target:after{
content: 'I am hovering';
border-radius: 5px;
background: #c0392b;
margin-left: 35px;
text-align: center;
padding: 5px 10px;
opacity: 0;
transition: all 1.5s ease;
}.hoverPE-target{
cursor: pointer;
margin: 0px 15px;
}.hoverPE-target:hover.hoverPE-target:after{
opacity: 1;
margin-left: 10px;
}<div class=”content”>
<div class=”hoverPE-target”>HOVER ME</div>
</div>
Note: CSS3 introduced the
::after
notation (with two colons) to distinguish pseudo-classes from pseudo-elements. Browsers also accept:after
, introduced in CSS2.
:Checked

.input-checked{
background: #3498db;
height: 20px;
font-size: 15px;
padding: 5px;
border-radius: 5px;
opacity: 0;
transform: translateX(40px);
transition: all 1.5s ease;
}.input-cBox:first-child:not(:checked) +
.input-radio:checked +
.input-cBox:checked +
.input-checked{
opacity: 1;
transform: translateX(0);
}<div class=”content”>
<input class=”input-cBox” type=”checkbox” checked>
<input class=”input-radio” type=”radio”>
<input class=”input-cBox” type=”checkbox”>
<div class=”input-checked”>Conditions are True</div>
</div>
We make a condition where the inputs should be checked except for the first child checkbox of .input-cBox
.
Here are the steps to make a conditions using :checked
- Target
.input-cBox
and.input-radio
using Adjacent sibling+
- Add
:checked
to.input-cBox
and.input-radio
except for the first child of the.input-cBox
- Then, target the first child of
.input-cBox
using:first-child
and add the condition:not(:checked)
.
If the conditions are true the style will work.
.input-cBox:first-child:not(:checked)
means that select the first child of.input-cBox
that is not checked.
:Target

.target{
font-size:18px;
opacity: 0;
transform: translateX(40px);
transition: all 1.5s ease;
}.target:target{
opacity: 1;
transform: translateX(0);
}<div class="content">
<a href="#element-target">Click Me</a>
<p class="target" id="element-target">Target is Set</p>
</div>
We can also target a specific element based on the fragment identifier / hash mark #
in the URL. When we click on the link <a href=”#element-target”>Click Me</a>
it will change the URL to https://example.com#element-target
.The #element-target
in the URL refers to the element with an id=”element-target”
, using :target
we can be able to set the style of an element that matches the current URL fragment identifier / hash mark #
in the URL.
.target:target
we add a class in thep
to set its style and specificity weight.
If we use the#element-target
to set thep
style, the:target
style will not work since using id in styling will have a higher specificity.
:link | :visited | :hover | :active (Links States)
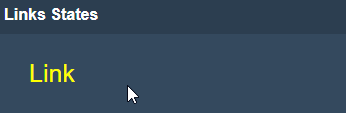
a:link{
color:yellow;
}a:visited{
color:green;
}a:hover{
color:red;
}a:active{
color:blue;
}<h4>Links States</h4>
<div class="content">
<a href="#link">Link</a>
</div>
Have you ever wondered why your links states style are not working?
Although you are thinking that they have same specificity weight, these is because links states have a specific trigger to set it’s style.
To make our links states all working, we need first set the style of :link
and then set :visited
style, :visited
style can be set before or after the style of :link
even that it will not override the style of :link
since :visited
style will be trigger when link is visited and :link
style will trigger when link is unvisited that’s why we set first the :link
because the default state of the link is unvisited. And then set the style of :hover
and set the :active
style at last because you need to trigger first the :hover
style before you can click to trigger the :active
style.
Note: When element have multiple style and these styles are equal specificity weight the last style that is set will be applied.
That’s why we need to set the style of :active
at the last. Also the :hover
and :active
style should come after :link
and :visited
styles so it will not override the style because you need to trigger the link to be visited or unvisited before you can trigger the :hover
and :active
style. With this we can came up to an idea of LVHA-order style to implement link specificity.
So to easily remember this link specificity, just remember LOVE (LV) and HATE (HA) or the LVHA acronym. You should also know why and how these link specificity or LVHA-order came up.
:focus
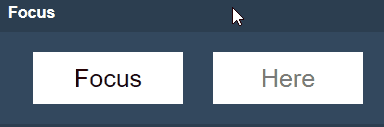
input:focus{
background: #2c3e50;
color: yellow;
}<div class="content">
<input type="text" value="Focus">
<input type="text" placeholder="Here">
</div>
We can trigger the focus on a element by clicks, taps or pressing the keyboard’s “tab” key. This is commonly used when we are filling out forms. Elements that can be focused are <a>
, <button>
, <input>
, <textareas>
, contenteditable
and tabindex
attributes.
It is also important that we should style our :focus
element to help a person know which element has the keyboard focus. Also, :focus
is in the Web Content Accessibility Guidelines(WCAG) 2.0 - Success Criterion(SC) 2.4.7 states that any keyboard operable user interface has a mode of operation where the keyboard focus indicator is visible. Like @rbottomley said that these days, with stricter accessibility requirements we should always remember to make our website content more accessible.
CSS Pseudo-Classes can do a lot of cool things.
You can also try and explore more on Pseudo-Class, like example using :hover
you can make a zoom effect on image when you hover on it or using :target
to create a modal and use fragment identifier to set the state of the modal. You can see the live code in this link.
Please do hit the CLAP button on the left, If this CSS tips and tricks helped you.
Thanks for your time reading my article.
Hope that this article helped you in your journey on Web Development.
If you have enjoyed reading this article, show support by buying me a coffee. I would really appreciate it.
Follow me on my links:
Instagram | LinkedIn | Stackoverflow | CodePen | Medium
✉️ Subscribe to CodeBurst’s once-weekly Email Blast, 🐦 Follow CodeBurst on Twitter, view 🗺️ The 2018 Web Developer Roadmap, and 🕸️ Learn Full Stack Web Development.