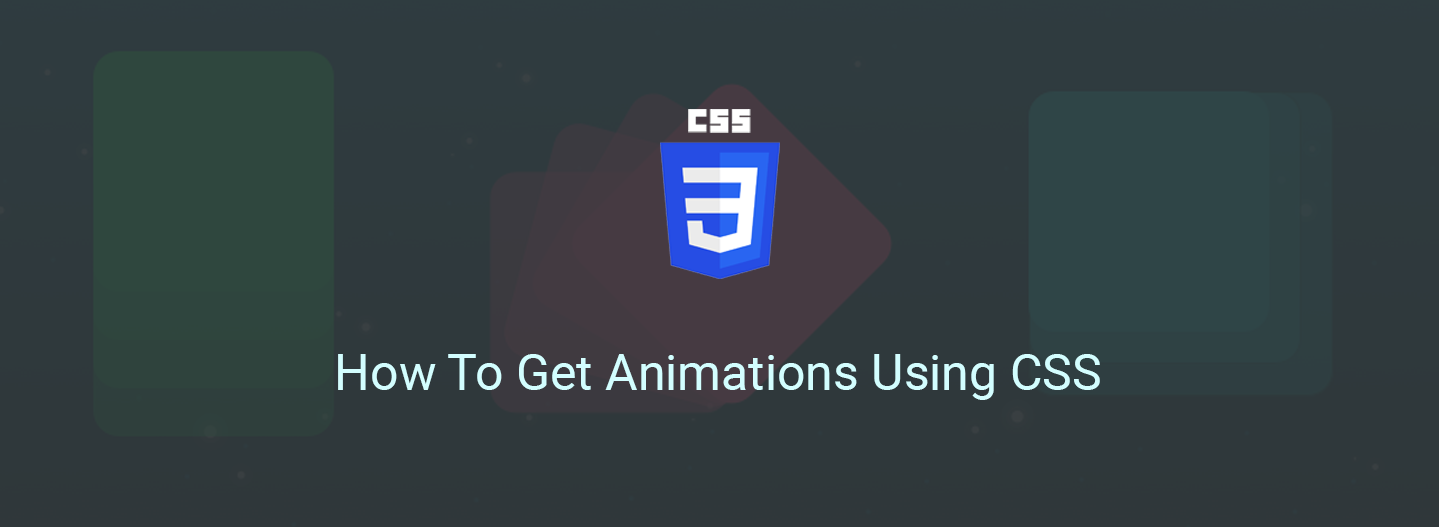
One might might wonder,
Why should we use CSS to get basic animations, when better animations could be achieved by using something like, say JavaScript?

One of the important reasons for using CSS for animations is that today’s browser are way better than the ones that we had a decade ago. This gives developers have enormous support and resources from the browser’s side to easily achieve things that were thought to be complex at one point in time.
To a non-developer, animations may seem to be something that is out of there reach, because most of them do not know how the properties of animations even work.
CSS being able to create basic animations can be see as a starting point for any amateur who wants to create websites that provide better user experience.
In this post, I will be be talking about how one can get animations using just CSS and simple shapes like squares, triangles, and circles.
@keyframes
@keyframes
is the main component of CSS animations. It is THE CSS rule where animations are created.
To make CSS animations work, @keyframes
is first defined and then bound to a selector.
Suppose you want to create an animation where you gradually change color from red to blue. We can divide this animation into various stages, so that color transitions between red, orange, yellow, green and blue.

@keyframes
has the following properties:
- Name: of our choice.
- Stages: 0%-100%.
- CSS styles: The style that you would like to apply for each stage.
The code here applies a transition to the opacity of an element, from opacity: 1 to opacity: 0. Each of the approaches above will achieve the same end result.
@keyframes fade {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
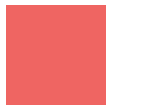
The Animation
The animation
property is used to call @keyframes
inside a CSS selector. animation can have multiple properties like:
animation-name
:@keyframes
name (remember we chose fade).animation-duration
: the timeframe length.animation-timing-function
: sets the animation speed.animation-delay
: the delay before our animation will start.animation-iteration-count
: how many times it will iterate.animation-direction
: gives you the ability to change the loop direction.animation-fill-mode
: specifies which styles will be applied to the element when our animation is finished.
Using this, our code would now look like this:
.element {
animation-name: fade;
animation-duration: 4s;
animation-delay: 1s;
animation-iteration-count: infinite;
animation-timing-function: linear;
animation-direction: alternate;
}
Or in short:
.element {
animation: fade 2s 1s infinite linear alternate;
}
Adding Vendor Prefixes
This is actually very important when we add CSS animations. Say you have designed a website full of animations and other stuff that gives a rich user experience. You open it on Chrome and Voila! It works perfectly! But when you open it on Firefox, the animations have crashed!
To avoid this from happening, a developer needs to add vendor prefixes.
The standard prefixes applied are:
- Chrome & Safari: ~webkit~
- Firefox: ~moz~
- Opera: ~o~
- Internet Explorer: ~ms~
Our code will now look like this:
.element {
-webkit-animation: fade 4s 1s infinite linear alternate;
-moz-animation: fade 4s 1s infinite linear alternate;
-ms-animation: fade 4s 1s infinite linear alternate;
-o-animation: fade 4s 1s infinite linear alternate;
animation: fade 4s 1s infinite linear alternate;
If we add @keyframes
,
@-webkit-keyframes fade { /* your style */ }
@-webkit-keyframes fade { /* your style */ }
@-webkit-keyframes fade { /* your style */ }
@-webkit-keyframes fade { /* your style */ }
@keyframes fade { /* your style */ }
Multiple Animations
To add multiple animations, just use a comma separator! 😄
@keyframes fade {
to {
opacity: 0;
}
}
@keyframes rotate {
to {
transform: rotate(180deg);
}
}.element {
animation: fade 2s 1s infinite linear alternate,
rotate 2s 1s infinite linear alternate;
}
Square To Circle
Let’s create a simple shape transition; a square to circle animation using the above principles. We will have five stages in total and for each stage we will define a border-radius, a rotation and a different background color to our element.
Basic Element
First, let’s create the markup, an element to animate.
<div></div>
Then, using an element selector, add default styling to the div:
div {
width: 200px;
height: 200px;
background-color: coral;
}
Declaring the Keyframes
Now let’s prepare @keyframes
, which we’ll call square-to-circle
, and the five stages
@keyframes square-to-circle {
0%{}
25%{}
50%{}
75%{}
100%{}
}
Let’s begin by adding the border-radius for each corner of the square:
@keyframes square-to-circle {
0% {
border-radius: 0 0 0 0;
}
25% {
border-radius: 50% 0 0 0;
}
50% {
border-radius: 50% 50% 0 0;
}
75% {
border-radius: 50% 50% 50% 0;
}
100% {
border-radius: 50%;
}
}
Additionally, we can also declare a different background-color and rotation for each stage.
@keyframes square-to-circle {
0% {
border-radius:0 0 0 0;
background:coral;
transform:rotate(0deg);
}
25% {
border-radius:50% 0 0 0;
background:darksalmon;
transform:rotate(45deg);
}
50% {
border-radius:50% 50% 0 0;
background:indianred;
transform:rotate(90deg);
}
75% {
border-radius:50% 50% 50% 0;
background:lightcoral;
transform:rotate(135deg);
}
100% {
border-radius:50%;
background:darksalmon;
transform:rotate(180deg);
}
}
Apply the Animation
div {
width: 200px;
height: 200px;
background-color: coral;
animation: square-to-circle 2s 1s infinite alternate;
}
One Last Thing
All works well in modern browsers, but Firefox renders transforming objects poorly. Just take look at these jagged lines:

To solve this issue add a transparent outline
to your div
and Firefox will now render things perfectly!
div {
...
...
...
outline: 1px solid transparent;
}
That’s All Folks!
That’s it! We’ve used CSS Animation syntax to create a simple, repeating animation.

I am Rajat S, a DC Comics fan and occasional ⚽️ player who is currently at GeekyAnts as Technical Content Writer.
Thanks to aditya thakur, who works as a Developer at GeekyAnts, for helping me with this post.
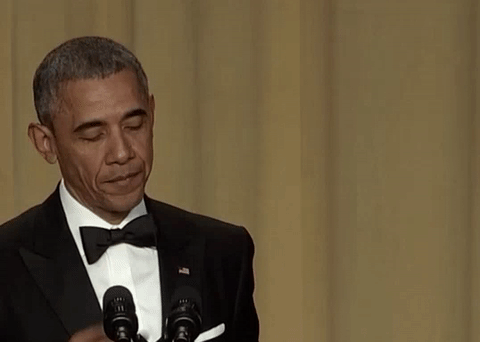
And thanks to you for reading this post! Please do 👏 and follow me if you liked this post!
✉️ Subscribe to CodeBurst’s once-weekly Email Blast, 🐦 Follow CodeBurst on Twitter, view 🗺️ The 2018 Web Developer Roadmap, and 🕸️ Learn Full Stack Web Development.