
How to Build a Cross-Browser New Tab Apps Using React
Building cross-browser new tab apps using React…
React is a declarative, efficient, and flexible JavaScript library for building smart user interfaces. React makes it painless to create interactive frontends for existing software.
You can take advantage of React features and build cross-browser new tab apps that can work on Chrome, Firefox, and other Chromium browsers.
New tab app or page is basically the page that comes up when you open a new tab on your browser or open your browser.
So in this article, I will explain how to build a new tab app by building an app called MediumTab. This app basically curates recent articles from Medium.com.
Note: At the time of writing I have only tested with chrome and firefox.
You can clone directly and see it in action on your browser: https://github.com/CITGuru/mediumtab
Setting Up The React App
To get started, we need to use a boilerplate to create our react app using create-react-app to avoid the react stressful setup and install some modules from npm.
Create and set up the app:
Install create-react-app and create medium-tab:
npx create-react-app medium-tab
or
npm install -g create-react-app
create-react-app medium-tab
cd medium-tab && npm start
Install npm modules needed:
yarn add axios semantic-ui-react semantic-ui-css
Set up the React app as an extension:
When you created the react app using create-react-app, a manifest.json file is added to the public folder. We’ll be updating that for our browser manifest file with the code below:
{
"name": "MediumTab",
"description": "Medium articles, the good way!",
"version": "1.0",
"manifest_version": 2,
"permissions" : ["management", "history", "storage"],
"chrome_url_overrides" : {
"newtab": "index.html"
},
"content_security_policy": "script-src 'self' 'sha256-GgRxrVOKNdB4LrRsVPDSbzvfdV4UqglmviH9GoBJ5jk='; object-src 'self'"
}
After updating the file, the next thing to do is to eject the app, build the app and add it to your browser. We’ll be using Chrome browser as an example in this tutorial.
By ejecting the app from create-react-app, we are able to customize our minimized scripts is being used and not violate the CSP rules.
Run npm run eject
or yarn run eject
to eject your app from create-react-app, then run npm install
to install the new modules added to your package.json file.
Openwebpack.config.js
file in the generated config folders, find the output
entry, under filename
change the static/js/[name].[contenthash:8].js
to static/js/app.js
and under chunkFilename
change the static/js/[name].[contenthash:8].chunk.js
to static/js/[name].chunk.js
or edit as below images:
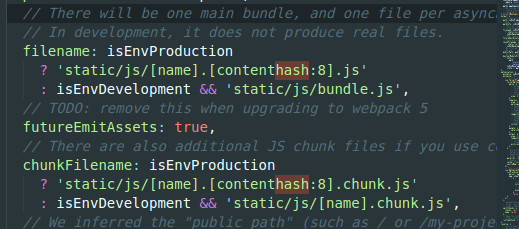

Run yarn build
or npm build
to build the app, which then generates a build folder for our built app.
In Chrome browser, go to chrome://extensions
page and turn on developer mode. This enables the ability to locally install a Chrome extension.
Now click on the LOAD UNPACKED
and browse to build
folder of app, this will install the React app as a Chrome extension.
That’s all, we have successfully created our first browser new tab application. Open a new tab and you will see something like below:

Notes: I am currently open to a remote Frontend Engineer position. If your team needs a remote frontend engineer, I am your guy. Don't hesitate to contact me: citguru.github.io
Building the React App
The next thing we need to do now is to build a simple app that curates the latest top ten articles from Medium. I built a RESTful API with Python/Django called Medrum that returns recent articles on medium randomly or base on the categories you requested for.
In your src
folder, create a file called Medium.js
and copy the below code:
We are directly pulling the articles from Medrum, then map the data into the Semantic Card components.
The above function pulled scraped articles from medrum using the axios http client library and put the returned data in our state component if it's successful. loading
is used to show if the http request is still not done and data not ready while noInternetError
is to show if there’s an error while sending the http request.
selectCategory = (e) => {
this.getMediumArticle(e.target.innerText)
}
We use this here to select the category and used that to pull category based articles from medrum.
componentDidMount(){
this.getMediumArticle()
}
This call the getMediumArticle()
when the app is being mounted.
We first of all check if the axios http request is still loading and return a loading component if so. Then we also check if there’s internet error and return internet connection error component.
Now head over to the App.js
file in the src folder and replace its content with below:
import React from 'react';
import './App.css';
import 'semantic-ui-css/semantic.min.css'
import Medium from './Medium';function App() {
return (
<div className="App">
<Medium />
</div>
);
}export default App;
Next, open the App.css
file in the src folder and replace it with the below:
Now lets try rebuilding the app using npm run build
and reloading our extension on Chrome.
You should see something like below:

That’s all.
If you enjoyed this article, ensure you clap and share.