How to Lazy Load features using React and Webpack
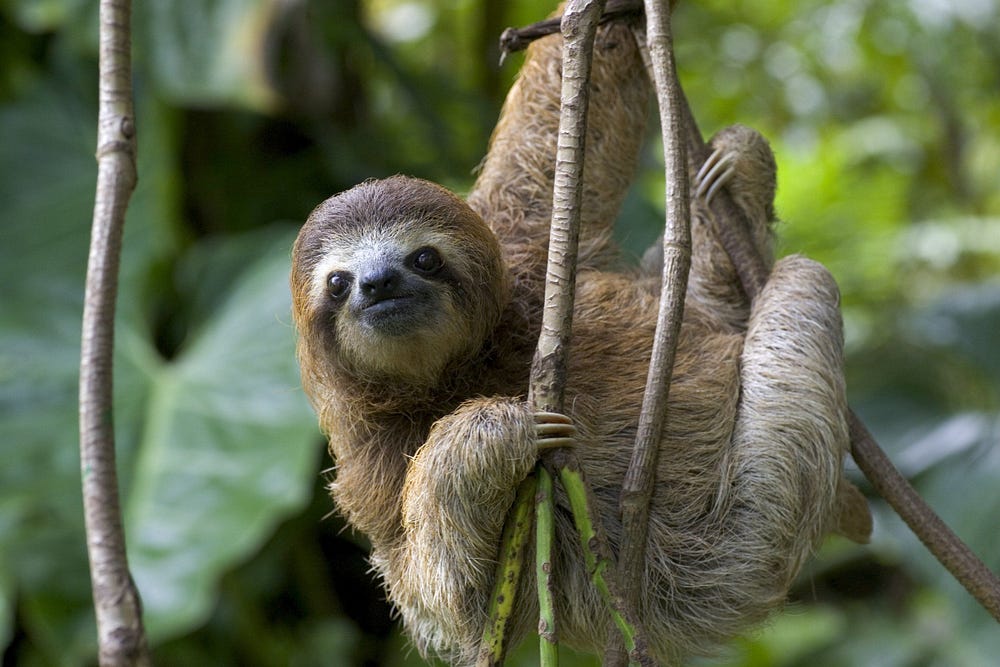
In the modern web, every kilobyte matters. We don’t want to load our app’s whole component library when user enters our site. We need to load components on demand, and after initial load, cache them in the memory for further use.
Also we want lazy loading logic to be separated from the component implementations.
We can solve this problem using Webpack import
functions and Higher order components.
Our HOC takes two arguments, second is the component which will be shown while component is loading, because we don’t want our users to be looking at the blank screen.
First argument is the getComponent
function, this is where we call Webpack import
:
One might ask why we don’t pass the path directly to the HOC. That’s because, Webpack needs this information statically (in compile-time). E.g. if we do something like this: import('./src/foo-${someVariable}.jsx')
Webpack will bundle all the files in the ./src
directory, which follow the pattern foo-*-jsx
. If we pass the whole string directly import('./src/foo-bar.jsx)
, it will only bundle this one file. But we cannot do this: path => import(path)
, because Webpack won’t know which files to consider, it won’t have any pattern to match files against.
So our HOC, calls import
before it mounts and displays the spinner while component is being downloaded.
This way we can easily convert any statically imported component into lazy-loadable one.
The above example works for Webpack 2 and newer, for Webpack 1 you could use require.ensure
API with the same approach.