Instagram Filters with JavaScript (p5.js)
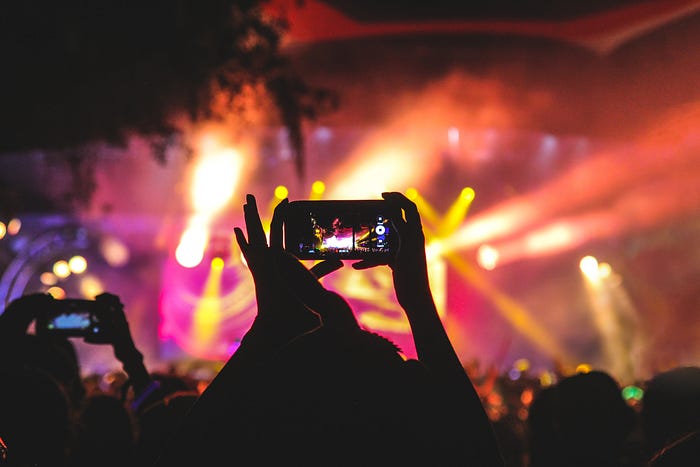
This tutorial is based on a workshop given by Cassie Tarakajian at CC Fest LA in 2017 and works best using Google Chrome on a computer with a webcam. Since this tutorial is web-based, there is no need for a cell phone.
Pressed for time? Skip right to the starter code and start customizing your filter!
Getting Started
- Open a new window in Google Chrome and go to the p5.js editor. You should see code that looks like this:
function setup() {
createCanvas(400, 400);
}function draw() {
background(220);
}
2. Make a variable. You are going to be creating a video capture using your computer’s camera. We need to store the video capture in a variable. At the very top of your code (before the functions), add in this code:
var capture;
This code declares a variable named capture
, but we haven’t put anything in it yet.
3. Set up your video capture. We need to store your computer camera’s video capture in the capture
variable. Add the following code in the setup
function right below the createCanvas
line, but inside the curly braces:
capture = createCapture(VIDEO);
capture.size(400, 300);
noStroke();
The size
specification makes the capture appear nicely proportional on your 400x400 canvas. You can play around with other sizes, but the image may be skewed or cut off!
4. Test the video capture. If you press the “run” button (the triangle shape that looks like a “play”) button, your video should appear below the grey canvas! Test it out. Google Chrome should ask permission in order to access your video.


If your video is not working, make sure you are using the Google Chrome browser. If you are using Google Chrome and your video is not working, team up with a friend whose camera is working.
5. Load all the pixels from the video. We need to load in the pixels of the video capture so we can start adjusting the colors. Add this code into your draw
function (between the curly braces) underneath where it says background(220);
:
capture.loadPixels();
6. Loop through all the pixels. Because there are so many pixels in the video capture, we can’t load all of them (the program will freeze), so we are going to load every fourth pixel. Add this code below the capture.loadPixels();
line to loop through the pixels:
var stepSize = 4;
for (var x = 0; x < capture.width; x += stepSize) {
for (var y = 0; y < capture.height; y += stepSize) {
var index = ((y*capture.width) + x) * 4;
// The code for your filter will go here!
}
}
Don’t worry to much about the details of the code. Here’s how it basically works:
First, we declared that the stepSize
of our filter would be four, meaning that we are only printing every fourth pixel. Then we use a construction called a for
loop to loop through the pixels using their x
and y
values. Then we get the index
of the current pixel using its (x, y) coordinates.
7. A word about pixels. Each pixel has a color that is a mix of red, green, and blue values. The way Instagram filters work is they detect the amounts of red, green, and blue in each pixel and alter these values. We are going to do the same thing!
8. Get the red, green, and blue values for each pixel. These values are listed consecutively for each pixel. Add this code right after you declare the index
variable inside the two loops:
var redVal = capture.pixels[index];
var greenVal = capture.pixels[index + 1];
var blueVal = capture.pixels[index + 2];
fill(redVal, greenVal, blueVal);
ellipse(x, y, stepSize, stepSize);
What happened here? We gathered the red, green, and blue values for each pixel and projected them onto the screen! Run the code, and you should see yourself on the camera again — but this time on the grey canvas, and slightly lower quality.
Soon, we will compensate for that low quality by putting our image through our own snazzy filters that we will code ourselves!
Starter Code
By now, you should have the following starter code:
var capture;function setup() {
createCanvas(400, 400);
capture = createCapture(VIDEO);
capture.size(400, 300);
noStroke();
}function draw() {
background(0);
capture.loadPixels();
var stepSize = 4;
for (var x = 0; x < capture.width; x += stepSize) {
for (var y = 0; y < capture.height; y += stepSize) {
var index = ((y*capture.width) + x) * 4;
var redVal = capture.pixels[index];
var greenVal = capture.pixels[index + 1];
var blueVal = capture.pixels[index + 2];
fill(redVal, greenVal, blueVal);
ellipse(x, y, stepSize, stepSize);
}
}
}
Customizing Your Filter
Now you can adjust the colors to create your own filter!
- Change the colors. You can edit how the colors appear in the line in the
draw
function that starts withfill
. The variablesredVal
,greenVal
, andblueVal
are actually numbers.
For instance, if I wanted to increase the amount of red in the image, I would multiply redVal
by two:
fill(redVal*2, greenVal, blueVal);
And if I wanted to decrease the amount of blue, I would divide blueVal
by two:
fill(redVal, greenVal, blueVal/2);
Even though web colors are red, green, and blue (slightly different from the primary colors you learned in art class), some of the rules about color mixing still apply. For instance, red and blue still make purple.
Play around with different color values and see what you can make!
2. Play with the stepSize. Change it to different numbers (the program will crash if you go too low) or do something crazy. Here’s how to make the stepSize correspond to the x-position of your mouse — instead of var stepSize = 3
, write:
var stepSize = floor(map(mouseX, 0, width, 5, 20));
3. Play with the stroke. What happens when you delete the line noStroke();
from the setup()
function?
4. Change the shapes. In the last line of code in the draw()
function, what happens when you change ellipse
to rect
?
Take a screenshot of filters you like! If you want to save your code, copy and paste it into a text editor so you don’t lose it. Then you can create an account on the p5.js editor and paste the code back in to keep using it!