JavaScript Interview Questions: Promises
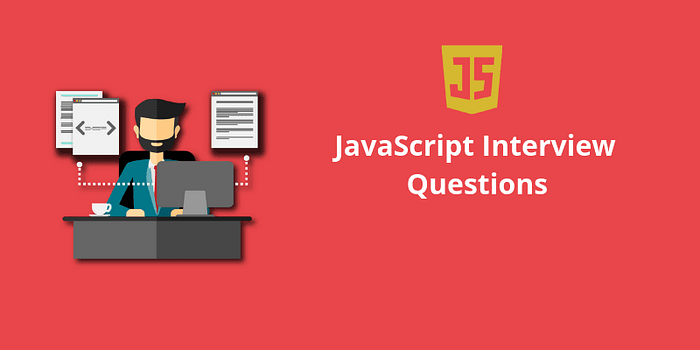
JavaScript has been the most used programming language for many years now, yet people continue to find it difficult to get a grasp of the language. This article will discuss some of the most frequently asked questions in JavaScript with the intention of helping readers overcome any confusion they may have around this language.
Question
What are the states of a promise?
Solution
- Pending
- Fulfilled
- Rejected
Question
Can we use await only with promises?
Solution
No, we can use await with promise as well as any object that implements a then function.
const thenable = {
then: function(callback) {
setTimeout(() => callback(2), 100);
}
};
const value = await thenable;
console.log(value); // 2
Question
What is the output of the following code?
const promise = new Promise((resolve, reject) => {
reject(Error('Some error occurred'));
})
promise.catch(error => console.log(error.message));
promise.catch(error => console.log(error.message));
Solution
Some error occurred
Some error occurred
You can add multiple handlers (catch, then, and finally) with a single promise.
Question
How can I write a sleep function using a promise?
Solution
function sleep(ms) {
return new Promise(res => {
setTimeout(res, ms);
});
}
Question
What is the output of the following code?
const promise = new Promise((resolve, reject) => {
reject(Error('Some Error Occurred'));
})
.catch(error => console.log(error))
.then(error => console.log(error));
Solution
Some error occurred
undefined
The catch function implicitly returns a promise, which can obviously be chained with a then. Since nothing is returned from the catch block, when error is logged in the then block it displays undefined.
Question
What is the output of the following code?
const promise = new Promise(res => res(2));
promise.then(v => {
console.log(v);
return v * 2;
})
.then(v => {
console.log(v);
return v * 2;
})
.finally(v => {
console.log(v);
return v * 2;
})
.then(v => {
console.log(v);
});
Solution
2
4
undefined
8
The finally block doesn’t receive any value, and anything returned from finally is not considered in the then block so the output from the last then is used.
Wrapping Up
In my personal opinion, while JavaScript isn’t the most complicated language it is a language with a lot of depth. If we can construct a clear understanding of the topics that we regularly use then it becomes extremely easy for us to get a better understanding of the nuances of JavaScript.