Structuring Business Logic in Node.js Application
Divide and conquer your application business logic
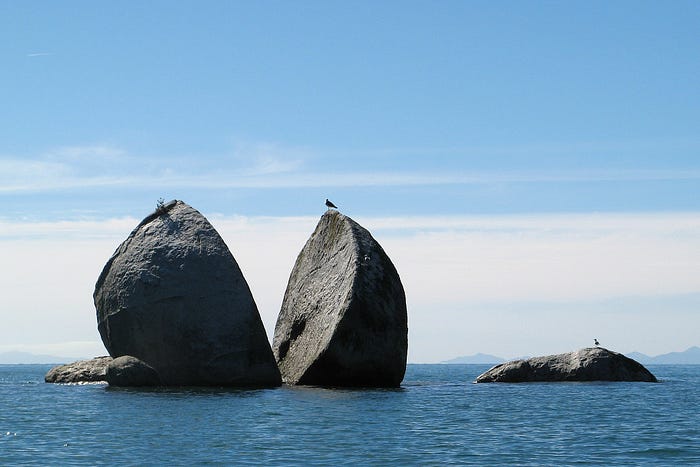
This blog post is about how one can organize business logic to follow the same module pattern popularized by Node.js as well as multiple frameworks that flourished on top of that allowing to achieve the goals with less lines of code, faster development and bring a higher level of performance and reliability to an application.
Why do we need frameworks?
The frameworks like express, hapi, marko, trooba have one common goal — free developers from reinventing the wheel and focus on implementing application business logic.
They provide guidelines and define how one can organize logic to handle different aspects of application such as handling incoming traffic, service invocations, rendering.
Putting a developer into simple and well defined constraints leads to predictable programming code, composed of simple, independent and testable components.
What is still missing?
Now if we take a medium sized app and look at its structure, we can see that it is usually organized using some of the above mentioned frameworks, but there is one place in many applications I have seen that is not yet structured. It is the business logic itself.
It is a wild world out there and the quality of it depends on developer’s skills. Most of the time it is mainly putting all application components together into a controller and initiating data retrieval; which may seem simple but again eventually can grow to an unmanageable monolithic piece of code.
Another important factor is testability of business logic. When all is put together it becomes a code that is not easy to test as all the components are hard bound. For example, when a developer wants to test how his app will react to 404 produced by one of the services, it requires an effort that many developers try to forgo, because it requires setting up the whole application and intercepting requests using nock; which works today and fails tomorrow as the service URL has changed. This leads to an untestable code that pulls other problems with it and leads to production bugs; which, as we all know too well, are more expensive to fix.
Why not apply the same rules to business logic to make simple, independent and easily testable business components or maybe we can call them business actions?
What? Another framework?
Now I would like to propose a solution that we have tested with a few application teams and their feedback was very positive. I would not call it a framework. It is a simple module, yet powerful enough to provide guidelines and structure to the business logic.
The module is called Oja, which means a flow or a stream in Estonian. It is based on a pub/sub pattern, but it is much more than that — it allows to convert your business logic into controllable action blocks and serves as a bus that achieves the following goals:
- Separation of concern by splitting business logic into independent testable components.
- Composition of more complex structures out of smaller business components while maintaining the same level of testability and independence.
- Remapping of streams into promises and events or vise versa or a mix of them depending on your requirements.
- Timeout support with status on what is still pending for easy troubleshooting. Many are familiar with the pain of a missing callback or a non-resolved promise that leads to a blank page in a browser or a timeout in service call.
- Cacheable events where oja allows to subscribe to the events that have already happened.
- Context propagation, especially valuable in asynchronous environments like Node.js.
Fun part: Coding
Let’s see how we can structure business logic on an imaginary frontend application.
Our application will be a web application based on express and will use standard MVC model. It needs to collect data from a few services, build a model and render page using some kind of template engine. The services return promises that provide async data.
Here’s high level view on the initial code when it is all setup in a page controller:
As you can see if I want to test what would happen with the application if sellerInfoSvc call fails, I would need to apply some effort to mock it, say, using nock for the service and provide mock data to all other services/operations, too.
This is challenging as the page controller code is monolithic and bound to actual service components; which limits the way I can test the code. Of course, I can make each component provide some kind of interface; which when activated will emit some mock data, but each developer can do it differently and the code still stays monolithic.
All of these makes an application developer uncomfortable writing tests and the developer tends to postpone this work until it is too late/complex and write incomplete basic positive test cases hoping QA will cover all of the rest.
Now, let’s see how we can split this logic into independent blocks that can be easily composed into more complex actions, tested separately and in composition.
We are going to use Oja module that provides an API to accomplish what we want.
Here’s what we will do
- Convert all services and operations like rendering or building a model into independent Oja actions.
- Compose all the actions into a page action and run it in the route handler.
- Each actions can consume data from other actions by topic.
- All actions start at the same time in parallel and there is no order of execution except the ones defined in each action by consuming specific topics from other actions.
- Provide tests demonstrating how actions can be tested separately and in composition.
Here’s example of sellerInfoSvc service before changes:
We can use it to define our sellerInfoAction:
Note: in case of error we do not want to propagate it as a general error as that would stop the flow completely, we wrap it into another object instead and publish under the same topic. With this setup, we can easily simulate service error responses and provide graceful degradation where it is possible.
The service action is independent from any external components. It is bound to the underlying service and only requires sellerId via consume statement.
Here’s how we can test the seller action in isolation:
Note: the framework does not enforce the way one defines topic names or data structures and assumes that the main usage for the framework is within one application domain and all the topics consumed by any component will be provided by other actions. Otherwise, it will fail/timeout and provide sufficient information on what is missing and needs to be fixed.
If you are ready to take it further, take a look at oja extension that provides type safety and schema for your data: https://github.com/fashion-js/fashion-model-action.
Now assuming we implement the other actions the same way, our page controller now should look like this:
Note: RenderAction abstracts the render logic, formatting and destination where the generated content should go.
RenderAction example:
The express route:
Note: Context is arbitrary and is just one of the many other ways to communicate the context information to all actions should they need it. In our case we wrap request and response to abstract our flow from where we get our parameters from and the output stream for our rendered content.
Here’s one example on how we can implement Context:
Now, let’s see how we can mock seller info service error:
That’s it for this blog post. The key point is to slice an application business logic into small functions that do not directly call other functions (hard binding), but consume and produce messages instead (soft binding).
I hope you got an idea on how you can split your business logic into independent pieces in a uniform way by using a simple pattern that will make your application code more manageable, testable and stable.
If you like to try it out, I have created a sample application that you can try here.