What is Pug.js (Jade) and How can we use it within a Node.js Web Application?
This quick tutorial will guide you through what Pug.js is, and how to use it with Node & Express in a real-world example.
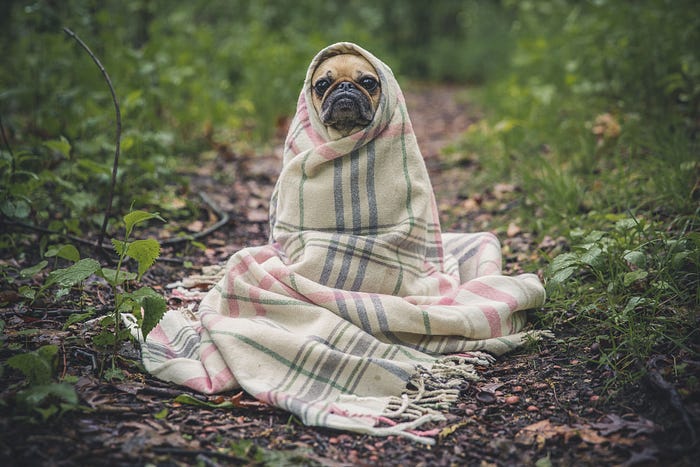
Prerequisites
In this tutorial, we’re going to be examining a weather website that I previously built with Node.js, Express JS, EJS, and OpenWeatherMap’s API. Here is the tutorial, and Here is the full code on GitHub. I strongly encourage you to check out those links before proceeding.
What is Pug?
To understand Pug, you need to remember that the browser reads HTML and CSS and then displays formatted images and text to the client based on what that HTML and CSS tells it to do.
Pug is the middleman.
Pug is a template engine for Node.js.
A template engine allows us to inject data and then produce HTML.
In short: At run time, Pug (and other template engines) replace variables in our file with actual values, and then send the resulting HTML string to the client.
How do we use Pug?
The same way we use other template engines.
Recall the following code from my: Build a Weather Website in 30 minutes with Node.js + Express + OpenWeather tutorial where we used EJS as our template language:
// TERMINAL
npm install ejs --save// SERVER.JS
app.set('view engine', 'ejs')
Using Pug is just as easy. Here are the three steps:
- Install Pug into your project:
npm install pug --save
- Set up your view engine:
app.set(‘view engine’, ‘pug’)
- Create a
.pug
file
So, what does a .pug file look like?
Great question! Before we look at a .pug
file, lets take a look at what our .ejs
file looked like in my last tutorial:
With EJS
Aaaaaand With Pug:
Pug is awesome
- Switching from EJS to Pug brought our code down from 27 lines to just 17!
- When you write with Pug, you write code that looks like paragraphs. This greatly improves code-readability and streamlines projects with multiple developers.
- There are no closing tags with Pug. Pug makes use of indentation to determine the nesting of tags. There are even shorthands for classes (.) and IDs (#)
- Most importantly, we can write JavaScript that actually (almost/kind of) looks like JavaScript within our pug files.
Pug is Bad
- With Pug, white-space matters. And it matters big time. The slightest mistake in your formatting/indenting/spacing means big problems for your code.
- With Pug, you can’t copy HTML from anywhere, you have to convert everything to Pug before you can use it.
Closing Thoughts
You can view the weather website full code (using Pug) on Github.
Hopefully, you can now understand what Pug.js is, what a .pug
file looks like, and why a template engine is important in web development. I publish a few articles and tutorials each week, please consider entering your email here if you’d like to be added to my once-weekly email list.
❤ If this post was helpful, please hit the little blue heart!
If tutorials like this interest you and you want to learn more, check out my Three awesome courses for learning Node.js, or my Best Courses for Learning Full Stack Web Development